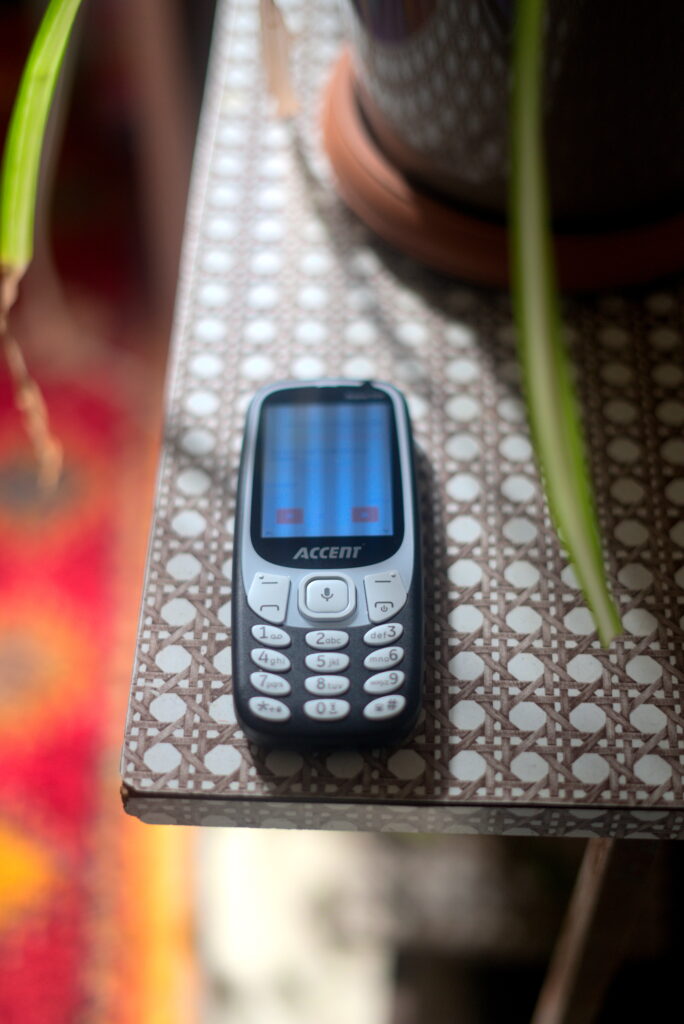
Flutter
Flutter is Google’s portable UI framework based on Dart language. It allows the deployment of web apps and native apps on iOS, Android, Windows, Mac, Linux and others using mostly the same source code.
(more…)Flutter is Google’s portable UI framework based on Dart language. It allows the deployment of web apps and native apps on iOS, Android, Windows, Mac, Linux and others using mostly the same source code.
(more…)ASCII art in PHP relies on the selection of a set of characters for the graphic representation. We didn’t find an optimal selection of characters mentioned anywhere. The example character sets seem to be chosen arbitrarily.
(more…)At jod.li, we started developing Flutter apps in June 2019 (9 months ago). We needed a cross-platform mobile development solution. We chose Flutter because it is evolving fast, yet, it is stable enough for robust development. There is a large community. Also, with Google as the underlying force behind, things are less likely to go wrong. Finally, we tried several other solutions without being convinced and sometimes even being very disappointed (several months of development on a non-Flutter solution and after an update, we ended up with an unrecoverable amount and complexity of compilation errors).
So, Flutter seemed like a good choice.
(more…)The purpose of those experiments was to determine whether an interlaced PNG image could be smaller in size than the equivalent non-interlaced PNG image.
(more…)I would like to know by advance the length of the uncompressed data within a PNG image whether it is encoded interlaced or not. Knowing and comparing the size of interlaced against non-interlaced PNG uncompressed (inflated) data can allow a wiser choice when encoding a PNG image and can also help while decoding. This can also explain why PNG images are usually greater when interlaced. All the following explanations are based on the PNG RFC. I focus on the cases when the pixel size is >= 8 bits.
(more…)